A Comprehensive Guide to C Programming Viva Questions: How to Prepare
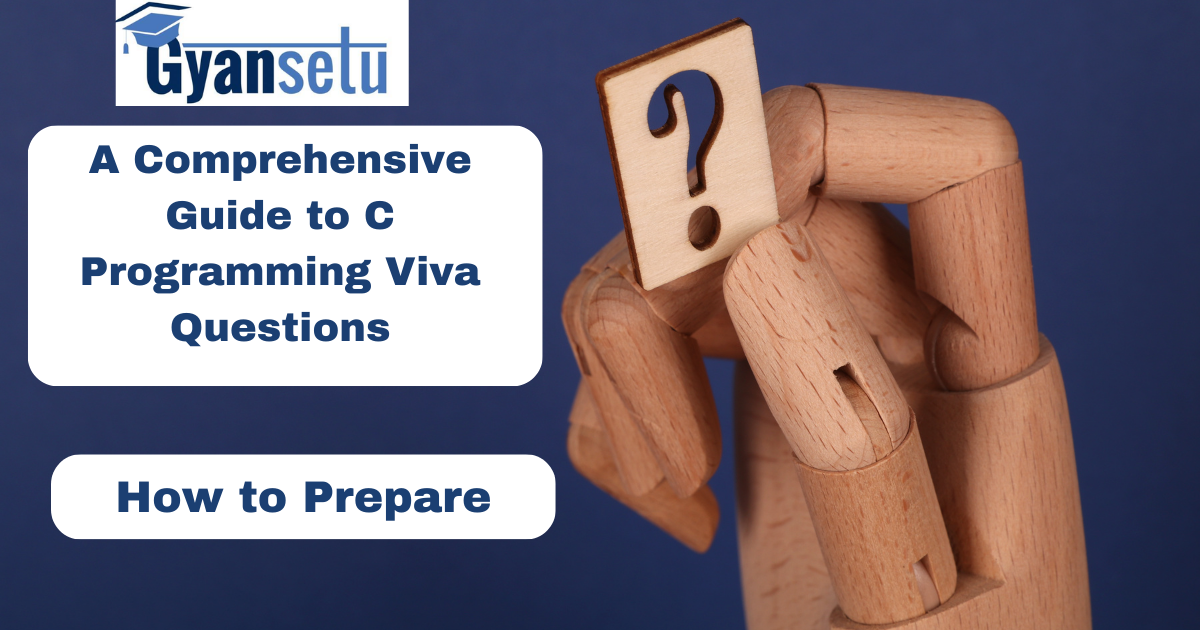
C programming is one of the world’s oldest and most prominent programming languages. The C programming language has several applications, such as in operating systems, language compilers, network drivers, assemblers, print spoolers, language interpreters, utilities, and databases.
This is why C programming viva questions have become integral to interviews in large tech-based MNCs. The programming language can be a bit complicated to learn. However, it is quite significant for enhancing your programming skills.
So, this article comprises the most asked C programming viva questions in interviews that will help you prepare for them.
1. What is C language?
Answer: It can be defined as a structure-oriented and mid-level programming language. It is also called the structured programming language. The programming language is a technique that facilitates the breakdown of large programs into small ones, where every module wields a structured code. This helps in minimizing mistakes as well as misinterpretation.
2. Why is C called a mother language?
Answer: C is often referred to as a mother language, as most JVMs and compilers are composed in C language. A lot of languages that were introduced later on borrowed substantially from it, such as Python, Javascript, Rust, C++, etc. It introduces some new major concepts, such as functions, arrays, and file handling, which are utilized in other languages.
3. Why is C called a mid-level programming language?
Answer: C is deemed a mid-level language because of its capacity to support both high-level and assembly-level or low-level features. The programming language can be wielded for writing menu navigated client billing and operating systems. Programs written in C language can be revised into low-level code, and therefore, they promote pointer arithmetic while also being high-level or machine-dependent.
4. Who founded the C programming language, and when was it founded?
Answer: Dennis Richie created the C programming language show around 1973 at Bell Labs Labs.
5. What are the basic Data Types supported in C Programming Language?
Answer: The Data Types supported by the C Language can be divided into 4 categories.
- Basic Data Types
These can be called the basic building blocks of data in the C programming language. They include:
Int – this is used for integers such as whole numbers.
Float- this is used for floating-point numbers that might have decimal parts.
Char- this is used for denoting individual characters, such as letters or symbols.
Double- this is similar to float but uses higher accuracy.
- Derived Data Types
These are created by integrating basic data types or derived types, for example:
Arrays – a collection of various elements having the same data type.
Pointers – this includes variables that compile memory addresses, allowing you to work with data delicately.
Structures – this includes custom data types holding various kinds of data under a single name.
- Enumerated Data Types
These are data types defined by users and comprise named integer constants. They are extremely useful for interpreting sets of relevant values.
- Void Data Type
The data type is wielded when a function doesn’t reciprocate any value or while working with pointers where a data type is unknown.
You can select the relevant data type to work with various kinds of information based on the task you have to perform.
6. What is the Dangling Pointer Variable in C Programming?
Answer: A Pointer in C Programming is wielded to indicate where the memory of a subsisting variable is located. Suppose that a specific variable is erased and the Pointer is nonetheless guiding towards the same memory location; then it is known as a Dangling Pointer Variable.
7. What do you understand by the Scope of the variable? What is the scope of the variables in the C programming language?
Answer: The portion of the code area that can be utilised for accessing the variables asserted in the program directly is known as the variable’s scope. Each variable retains a definite scope, and the variable is erased automatically beyond this area. Almost all identifiers are statistically or lexically scoped in the C programming language. To put it mildly, the scope of a variable is equivalent to its existence in the project.
8. What are the features of the C programming language?
Answer: Here are some key features of the C programming language.
- Portability
- Easy to expand
- Mid-level language
- Fast and productive
- General Purpose language
- Retains a rich functioning library
- Affluent set of in-built operators
- Structured language
- Modularity
9. What do you mean by static variables?
Answer: Variables in the C programming language that retain the data values between different function calls even when they are erased or out of their scope are called static variables. They can be employed repeatedly in the program without initialization.
These variables are designated a 0 initial value without initialization.
C
// C program to print initial
// value of static variable
#include <stdio.h>
int main()
{
static int var;
int x;
printf(“Initial value of static variable %d\n”, var);
printf(“Initial value of variable without static %d”,
x);
return 0;
}
Output
Initial value of static variable 0
Initial value of a variable without static 0
10. What are preprocessor directives in C?
Answer: In C programming language, preprocessor directives are deemed in-built predefined conditions that function as a command to the compiler and are performed before the execution of the program. Various steps are included in writing and implementing a program in the C programming language. Some crucial Preprocessor Directives are File Inclusion, Macros, Conditional Compilation, etc.
11. Differentiate between malloc() and calloc() in C.
Answer: Calloc() and malloc() are dynamic or active memory-assigning functions.
The header file used for facilitating the dynamic memory allotment in the C Programming language is “stdlib.h”.
Here are some major differences between Malloc() and Calloc().
- Malloc() creates a single block-sized memory, whereas the Calloc() function allocates memory of more than one block to a variable.
- The Malloc() function accepts one argument, whereas the Calloc () function accepts approximately two arguments.
- Regarding speed, the malloc () function is slower than the malloc() function. In addition to this, the malloc () function also procures lower time efficiency than the malloc() function.
12.What is the use of scanf() and printf() in C Programming Language?
Answer: printf() is used for printing specific values on the screen, and scanf() is employed for scanning the values. A reasonable data type format specifier is required for scanning and printing. Here are some examples:
- %s: This data type format specifier is used for printing and scanning the value of a string.
- %d : This data type format specifier is employed for printing and scanning the value of an integer.
- %f: This data type format specifier displays and scans a float value.
- %c: This data type format specifier is employed for displaying and scanning the value of a character.
13. Differentiate between Formal Parameters and Actual Parameters.
Answer: The parameters carried to the subdivided function by the main function are known as Actual Parameters, whereas the parameters asserted at the end of the Subdivided function are known as Formal Parameters.
14. What do you mean by a Nested Structure?
Answer: A structure that comprises one or more structural members is called a nested structure in a C programming language. The structure is a data type specified by the user for storing a collection of different data types as one data type, and the elements of a structure are called its members. Nested structures are very effective in organizing detailed data and can also help improve a program’s readability.
15. What are pointers, and state their features?
Answer: Pointers in C are used for storing the location or address of a variable, as well as for referring to different pointer functions. The primary objective of the pointer is to preserve memory space and improve the rate of performance. Some features of pointers are:
- It is used to pass statements by reference
- Execution of data structures
- To access array elements
- For returning considerable values
16. What is an array? Give an example.
Answer: An array in C programming language is a collection of specific-sized identical data items reserved in contiguous or adjacent memory locations. They can be wielded for storing primitive data types like float, int, char, etc., along with user-defined data types like structures and pointers.
For instance, a data of 200 integers had to be stored, an array can be created for it, like Int data [200].
17. Differentiate between the Compiler and the Interpreter.
Answer: Here are some differences between compiler and interpreter:
- High-level programming languages, such as Python, JavaScript, etc., use a compiler for translating source code into machine code before the program starts running. Interpreters translate source code into machine code for high-level programming languages line-by-line while the code runs.
- The time taken for analyzing sources is higher for compilers than interpreters, but the general execution of compilers is relatively faster when compared to interpreters.
Conclusion
These were some really important C programming viva questions, so make sure you prepare them for your next interview.